If you have an adafruit TTL Serial JPEG Camera you may have found that downloading an uncompressed 640×480 JPEG from the camera can take up to 15 seconds. Depending on your application this might be far too long!
Joined: Thu Jan 08, 2015 12:22 pm. Re: Problem with TTL Serial JPEG Camera and Arduino Yun. By adafruitsupportrick on Sat Jan 10, 2015 10:41 am. You can't use pins 2 and 3 on the Yun for software serial. Instead, connect the camera TX to pin 8 and the camera RX to pin 7. TTL Serial Camera I am using an Arduino Uno and I am trying to hook up a Camera to the RX and TX input/output. In the code for testing the camera, they use Serial1.begin. Connect your TTL Serial Camera module with the Arduino and Ethernet Shield as shown in the diagram below. Here, we will use two Arduino digital pins and a Software Serial port to communicate with the camera. Arduino Security Camera with Motion Detection; Prerequisites; Getting started with TTL Serial Camera; Connecting the TTL Serial Camera with Arduino and Ethernet Shield; Uploading images to Flickr; Summary; 5. Solar Panel Voltage Logging with NearBus Cloud Connector and Xively.
Camera compatible with Arduino (ideally with IR LEDs and motion detection built in. Adafruit's TTL weatherproof serial camera) $55 SD Card ( Adafruit breakout board with micro SD ) $7.50 LCD matrix for displaying status ( 2x16 Adafruit ) $10.
To speed things up a bit you can add compression to the image. Adding up to 40% can decrease the download time for a picture to about 5 seconds but at the same time you have to sacrifice picture quality.
So how else can you speed up the transfer? How about upping the transfer speed!
The camera by default uses 38400 baud but it is capable of much more than this. The question though is how to change the settings?
The Arduino Uno can do up to 115200 baud so it seems like a good idea to me to set the camera to the same speed. This should improve the download times by a fair bit more. (At the present time I haven’t been able to test by how much.)
So how do you get the camera to run at this faster speed? adafruit provide a library for the Arduino that provides functions for virtually all the things you might want to use on the camera. Unfortunately, at present there isn’t a function for changing the baud. After doing a lot of hunting around the interwebs I found there were quite a few people wanting to change the baud but no answer on how to do it. I put my thinking hat on and after a few hours of experimenting I came up with the following test code…
#include <SoftwareSerial.h>

SoftwareSerial softSerial(2, 3); //Set Software serial to use Pins 2 and 3
char serInStr[100];
int serInIdx =0;
int serOutIdx =0;
void setup() {
Serial.begin(9600); //Set Hardware Serial to 9600 baud - For outputting debug messages
Serial.println('Start');
softSerial.begin(38400); //Set Software serial to 38400 baud - Default setting for the camera
Serial.println('Softserial Baud has been set to 38400');
delay(10); //Pause for 10ms to let it all settle down. Probably not needed!
Serial.println('Get Camera Version');
getCamVers(); //Call getCamVers function
Serial.println('Setting Camera Baud to 115200');
setBaudMax(); //Call setBaudMax function
Serial.println('Reset softSerial to 115200');
softSerial.end(); //Disconnect serial connection to camera
softSerial.begin(115200); //Reconnect serial connection to camera at 115200 baud
Serial.println('Get Camera Version again using 115200');
getCamVers(); //Check camera version again to prove everything is working at 115200
}
void loop() {
//Do nothing
}
void getCamVers() {
uint8_t ByteData[5]={0x56, 0x00, 0x11, 0x00}; //String of bytes that requests version number from camera
softSerial.write(ByteData,5); //Send string to camera
delay(10); //Pause to let the camera deal with the request
if(softSerial.available()){ //Check if serial buffer has a response from camera
while(softSerial.available()){ //Loop as long as the serial buffer contains data
serInStr[serInIdx] = softSerial.read(); //Read data from serial buffer. Copy into array
serInIdx++; //Increase array count by 1
}
}
for(serOutIdx=0; serOutIdx < serInIdx; serOutIdx++) { //Loop through array
Serial.print(serInStr[serOutIdx]); //Print each value in array to consol
}
Serial.println(); //Print end of line
}

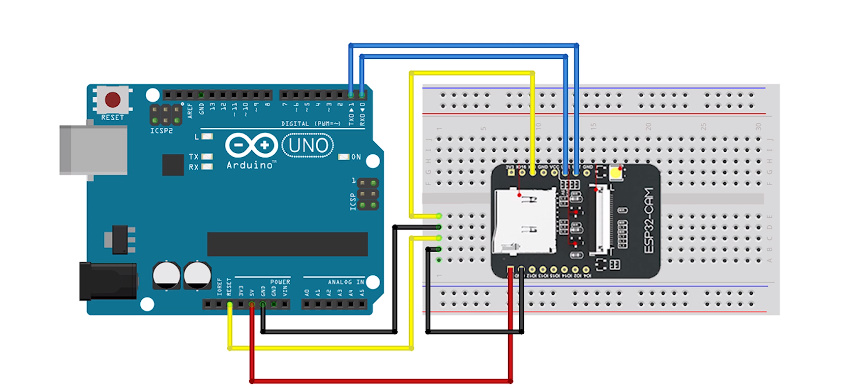
void setBaudMax() {
uint8_t ByteData[7]={0x56, 0x00, 0x24, 0x03, 0x01, 0x0D, 0xA6}; //String that sets baud to 115200
softSerial.write(ByteData,7); //Send string to camera
//Should really check for a suitable response here!
}
How to make your own spy cam!
- 27,318 views
- 5 comments
- 13 respects
Components and supplies
Apps and online services
|
About this project
in todays video I show you how to setting up esp cam with your own arduino.
full tutorial on my youtube channel
What is esp32 :
is a series of low-cost, low-power system on a chip microcontrollers with integrated Wi-Fi and dual-mode Bluetooth.
Subscribe to my youtube channel
Follow me on instagram
what is esp32-cam ?
The ESP32-CAM is a small camera module with the ESP32-S chip that costs approximately $10. Besides the OV2640 camera, and several GPIOs to connect peripherals, it also features a microSD card slot that can be useful to store images taken with the camera or to store files to serve to clients.
Subscribe to my youtube channel
Follow me on instagram
ESP32-CAM Specifications :
- The smallest 802.11b/g/n Wi-Fi BT SoC Module
- Low power 32-bit CPU, can also serve the application processor
- Up to 160MHz clock speed,summary computing power up to 600 DMIPS
- Built-in 520 KB SRAM, external 4MPSRAM
- Supports UART/SPI/I2C/PWM/ADC/DAC
- Support OV2640 and OV7670 cameras, Built-in Flash lamp.
- Support image WiFI upload
- Support TF card
- Supports multiple sleep modes
- Embedded Lwip and FreeRTOS
- Supports STA/AP/STA+AP operation mode
- Support Smart Config/AirKiss technology
- Support for serial port local and remote firmware upgrades (FOTA)
Subscribe to my youtube channel
Follow me on instagram
Subscribe to my youtube channel

Follow me on instagram
Code
Schematics
Author
CiferTech
- 2 projects
- 18 followers
Published on
October 10, 2019Members who respect this project
and 6 others
See similar projects